Introduction
As a freelance Laravel web developer, I get a chance to work on a wide variety of projects. These projects range from small applications, to large enterprise-scale applications, and even open-source projects. So I get to see a lot of different codebases and see how different teams tackle problems.
There is one thing that I see in almost every application, and that is getting the logged in user in a controller or request class. There's no one way to do this, and I've seen a lot of different approaches.
Out of pure curiosity, I put out a poll on Twitter to ask Laravel developers how they'd get the authenticated user in their own applications. I got 137 responses, so I thought it'd be fun to share the results in this Quickfire article so you can see how other developers are doing it.
The Poll
Here's the tweet that I posted:
How do you get your current logged in user in your Laravel apps?
— Ash Allen ๐ (@AshAllenDesign) April 21, 2023
I like using Option A! ๐#laravel #php pic.twitter.com/1ZJZlVnuo8
The Results
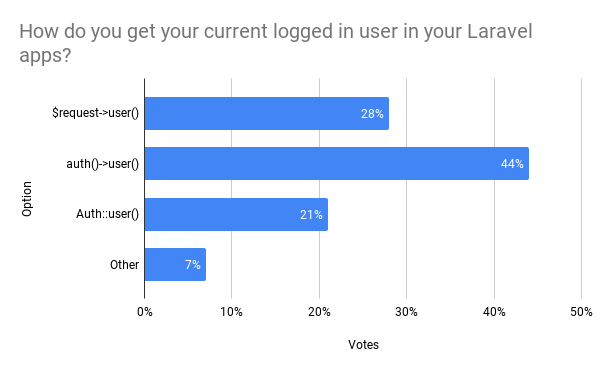
The votes were as follows:
- auth()->user() - 60 votes (44%)
- $request->user() - 39 votes (28%)
- Auth::user() - 29 votes (21%)
- Other - 9 votes (7%)
Analysis
auth()->user()
According to the poll, the most popular way of getting the current authenticated user in Laravel is by using the auth()->user()
helper function.
From reading the reasons behind this choice, it seems that this was popular because it doesn't require you to import any classes into your code, such as the Auth
facade or Request
class. Instead, you can just use the auth()
helper function directly in your code to get the authenticated user.
For example, in your controller, you could do the following:
1class UserController extends Controller2{3 public function show()4 {5 $user = auth()->user();6 7 return view('user.show', ['user' => $user]);8 }9}
Some people may argue that using this approach is bad practice because it's using a global helper function. Usually, I think I'd probably agree with this because I like to avoid using helper functions when possible and use methods in classes instead. However, in this case, I quite like using the auth()
helper function. It's short, simple, and easy to remember. I've also noticed that it's used in almost every Laravel project I've ever worked on, so it's a pretty common approach and I like to stick to the conventions that teams are already using.
The main danger that I see (and have seen many times) with this approach is that it's tempting to use it outside your controllers and request classes. For example, let's say that we have an action or service class that updates the details of the logged in user. The code might look something like this:
1class UpdateUserDetailsAction 2{ 3 public function execute(array $data) 4 { 5 $user = auth()->user(); 6 7 $user->update($data); 8 9 // ...10 }11}
In this case, we're using the auth()
helper function to update the user's details. But by doing this, we've now coupled our action class to the authentication. This means if we wanted to use this action class in a different context (such as updating another user's details), we wouldn't be able to do so with the current implementation.
The action class could be improved by passing the user to the method instead, like so:
1class UpdateUserDetailsAction2{3 public function execute(User $user, array $data)4 {5 $user->update($data);6 7 // ...8 }9}
This means now we could call the action class method like so:
1(new UpdateUserDetailsAction)->execute(2 user: auth()->user(),3 data: ['name' => 'Ash Allen']4);
So, in my personal opinion, I think as long as you remember to only use the auth()
helper function in your controllers and request classes, then it's a perfectly fine approach to use.
$request->user()
The second most popular way of getting the authenticated user in Laravel is by using the $request->user()
method. This method gets the current logged in user from the request itself.
I personally like using this approach the most. As I've already mentioned, I like avoiding global helper functions when possible, so I like that this approach uses a method on the Request
class instead. I also feel like it reads quite nicely and makes it harder to accidentally use it outside your controllers and request classes (unless you choose to access it using the request()->user()
function).
For example, in your controller, you could do the following:
1use Illuminate\Http\Request; 2 3class UserController extends Controller 4{ 5 public function show(Request $request) 6 { 7 $user = $request->user(); 8 9 return view('user.show', ['user' => $user]);10 }11}
You may have noticed that this approach also requires you to import the Request
class into your controller.
Auth::user()
The third most popular way of getting the authenticated user in Laravel is by using the Auth::user()
method.
Personally, I've never used this approach in my own code before because I've always either opted for the auth()->user()
or $request->user()
methods instead. However, I can see why some people might prefer this approach because it does look very "Laravel-y" with the use of the Auth
facade.
For example, in your controller, you could do the following:
1use Illuminate\Support\Facades\Auth; 2 3class UserController extends Controller 4{ 5 public function show() 6 { 7 $user = Auth::user(); 8 9 return view('user.show', ['user' => $user]);10 }11}
Other Approaches
There were also several other approaches that people mentioned in the poll. These included creating their own helper functions (such as authUser()
), using dependency injection to resolve a user from an interface, and using the request()->user()
helper function.
Although these approaches weren't as popular as the ones mentioned above, I think they're still worth mentioning because you may come across them when working on a Laravel project.
It's important to remember that these results are by no means a representation of the entire Laravel community. The poll was only open for 24 hours and was on Twitter. There's a possibility that these results could be skewed towards a certain type of developer. But I do still think they give a good indication of the approaches you'll see in different projects, even if the numbers aren't 100% accurate.
FAQ
How Do I Check if a User Is Logged In in Laravel?
There are a few different ways that you can check whether the current request was made by an authenticated user in Laravel. Let's take a look at some of the most common ways.
If you're using the Auth
facade or auth
helper, you can use the check
method to check whether the user is logged in. If the user is logged in, then it will return true
. Otherwise, it will return false
. For example:
1if (Auth::check()) { 2 // The user is logged in... 3} else { 4 // The user is not logged in... 5} 6 7if (auth()->check()) { 8 // The user is logged in... 9} else {10 // The user is not logged in...11}
The Auth
facade and auth
helper both also include a guest
method which can be used to check whether the request was made by an unauthenticated user. If the request was not made by an authenticated, then it will return true
. Otherwise, it will return false
. For example:
1if (Auth::guest()) { 2 // The user is not logged in... 3} else { 4 // The user is logged in... 5} 6 7if (auth()->guest()) { 8 // The user is not logged in... 9} else {10 // The user is logged in...11}
If you're using the Request
class, there isn't a check
method for you to use. However, you can use the user
method to check whether the user is logged in:
1if ($request->user()) {2 // The user is logged in...3} else {4 // The user is not logged in...5}
How Do I Get the Current Logged In User's ID in Laravel?
If you're using the Auth
facade or auth
helper, you can use the id
method to get the ID of the currently authenticated user. For example:
1$id = Auth::id();2 3$id = auth()->id();
If there is no authenticated user, both of these methods will return null
. Otherwise, they will return the ID of the authenticated user.
If you're using the Request
class, you can use the user
method to get the currently authenticated user. You can then use the id
method on the user to get the ID of the user. For example:
1$id = $request->user()?->id;
We are using the null safe operator (?->
) here to ensure that we don't get an error if there is no authenticated user. If there is no authenticated user, then the id
property will be null
. Otherwise, it will be the ID of the authenticated user.
Conclusion
Hopefully, this article has given you a quick insight into a few of the different ways that you can get the authenticated user in Laravel.
If you enjoyed reading this post, I'd love to hear about it. Likewise, if you have any feedback to improve the future ones, I'd also love to hear that too.
You might also be interested in checking out my 220+ page ebook "Battle Ready Laravel" which covers similar topics in more depth.
If you're interested in getting updated each time I publish a new post, feel free to sign up for my newsletter below.
Keep on building awesome stuff! ๐